Mastering JavaScript Objects
Introduction:
Welcome to the world of programming! In this tutorial, we will delve into the fundamental concept of JavaScript programming – Objects. Understanding objects is crucial for any JavaScript developer, as they play a pivotal role in organizing and manipulating data. By the end of this guide, you’ll have a solid grasp of JavaScript objects and be well on your way to becoming a proficient programmer.
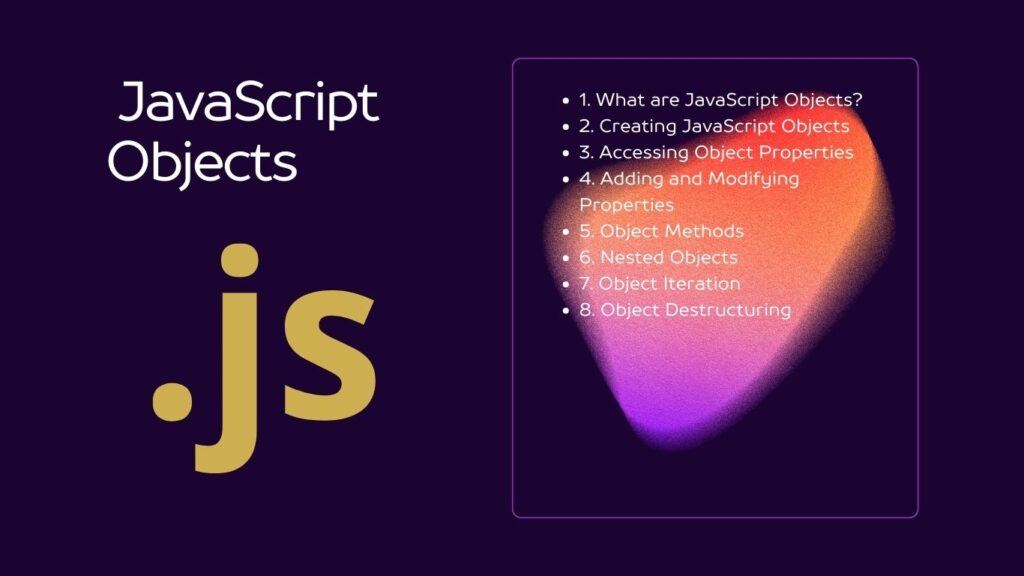
Table of Contents
1. What are JavaScript Objects?
JavaScript objects are complex data types that allow you to store and organize data using a key-value pair. Think of an object as a container that holds various pieces of information about a particular entity.
Example:
// Declaring an empty object
let person = {};
2. Creating JavaScript Objects
Objects can be created in various ways, but the most common is using object literal notation.
Example:
// Creating an object with properties
let person = {
name: 'John Doe',
age: 25,
profession: 'Developer'
};
3. Accessing Object Properties
You can access object properties using dot notation or square brackets.
Example:
// Accessing object properties
console.log(person.name); // Output: John Doe
console.log(person['age']); // Output: 25
4. Adding and Modifying Properties
Objects are mutable, meaning you can add or modify properties after creation.
Example:
// Adding a new property
person.location = 'Cityville';
// Modifying an existing property
person.age = 26;
console.log(person);
// Output: { name: 'John Doe', age: 26, profession: 'Developer', location: 'Cityville' }
5. Object Methods
Objects can also contain functions, known as methods.
Example:
// Adding a method to the object
person.sayHello = function() {
console.log(`Hello, my name is ${this.name}`);
};
// Calling the method
person.sayHello();
// Output: Hello, my name is John Doe
6. Nested Objects
Objects can be nested within other objects to represent more complex structures.
Example:
let company = {
name: 'TechCo',
employees: {
manager: 'Alice',
developer: 'Bob'
}
};
console.log(company.employees.manager); // Output: Alice
7. Object Iteration
You can iterate over the properties of an object using loops or methods like Object.keys
.
Example:
// Iterating over object properties
for (let key in person) {
console.log(`${key}: ${person[key]}`);
}
// Output:
// name: John Doe
// age: 26
// profession: Developer
// location: Cityville
8. Object Destructuring
Destructuring allows you to extract specific properties from an object.
Example:
// Destructuring object properties
const { name, age } = person;
console.log(name, age);
// Output: John Doe 26
9. Conclusion
Congratulations! You’ve now covered the basics of JavaScript objects. This knowledge is foundational for any JavaScript developer. Practice these concepts by creating your own objects and manipulating their properties. Stay tuned for more advanced topics in our future tutorials.
Now that you’ve mastered JavaScript objects, you’re ready to explore more complex JavaScript features. Happy coding!