Introduction To Python Classes
Welcome to our comprehensive guide on Python classes, where we will demystify this fundamental concept in the Python programming language. Whether you’re a complete beginner or looking to reinforce your understanding, this tutorial is designed to cater to all levels of expertise. Python classes are the backbone of object-oriented programming (OOP) and play a pivotal role in structuring code for real-world applications. In this article, we will start from scratch and gradually lead you through the intricacies of Python classes, from defining and creating classes to using inheritance and achieving polymorphism. So, if you’ve ever wondered how to harness the full power of Python classes, you’re in the right place.
Python classes are essentially blueprints that allow you to define your own data types with attributes and methods. They are the building blocks of most Python programs, enabling you to model and organize your code around real-world entities, making your code more readable and maintainable. As we delve into the world of Python classes, you’ll learn how to create classes, initialize objects, and access attributes and methods associated with them. Furthermore, we’ll explore the concept of inheritance, which empowers you to create new classes based on existing ones, promoting code reuse and extensibility. The art of polymorphism, where different objects can be treated as instances of a common superclass, will also be unveiled in this tutorial.
Python classes are not just a theoretical concept but a practical necessity in modern programming. They provide a structured approach to code organization, encourage reusability, and facilitate collaboration among developers. Whether you’re building a simple script or a complex software application, understanding Python classes is essential for mastering Python and writing efficient, maintainable, and scalable code. So, let’s embark on this journey into the world of Python classes, where you’ll gain valuable skills that can be applied to a wide range of programming projects.
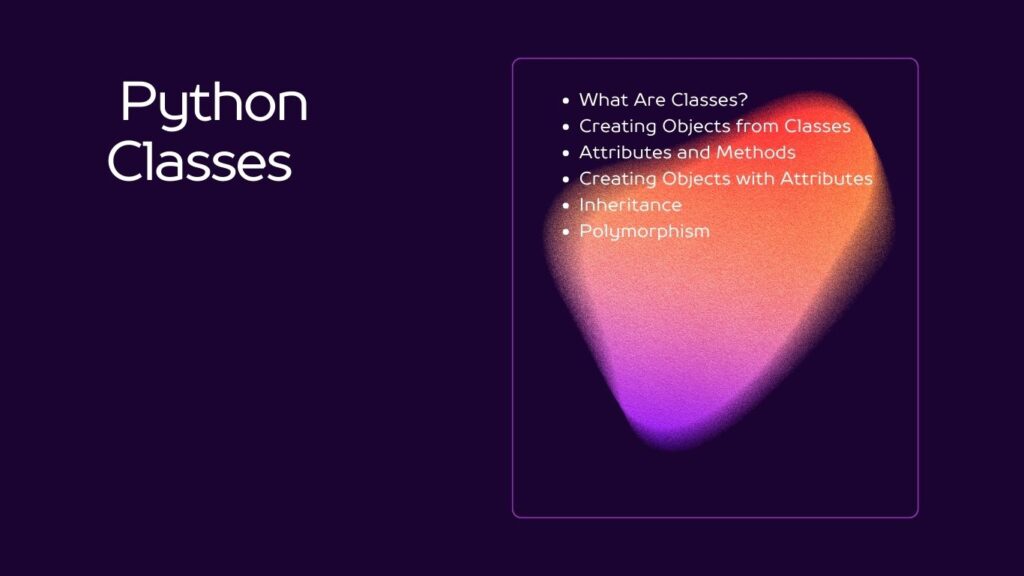
Table of Contents
Prerequisites
Before diving into Python classes, make sure you have Python installed on your computer. You can download Python from the official website (python.org). Basic knowledge of Python syntax will also be helpful.
What Are Classes?
In Python, a class is like a blueprint or template for creating objects. Objects are instances of a class, and they can have attributes (variables) and methods (functions) associated with them. To define a class in Python, you use the class
keyword.
# Define a simple class
class Dog:
pass
Creating Objects from Classes
Once you have a class, you can create objects from it. These objects will inherit the attributes and methods defined in the class.
# Create an instance of the Dog class
my_dog = Dog()
Attributes and Methods
Classes can have attributes (variables) and methods (functions). Let’s add attributes to our Dog
class and a method to make it more meaningful.
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return "Woof!"
- The
__init__
method is a special method called the constructor. It is executed when you create a new object. It initializes the object’s attributes. - The
bark
method is a simple method that returns a string.
Creating Objects with Attributes
Now, let’s create a Dog
object with attributes and call its methods.
# Create a Dog object
my_dog = Dog("Buddy", "Golden Retriever")
# Access attributes
print(my_dog.name) # Output: Buddy
print(my_dog.breed) # Output: Golden Retriever
# Call a method
print(my_dog.bark()) # Output: Woof!
Inheritance
In Python, classes can inherit attributes and methods from other classes. This concept is called inheritance. It allows you to create new classes based on existing ones, reusing their functionality.
class Bulldog(Dog):
def bark(self):
return "Woof! Woof!"
In this example, we created a Bulldog
class that inherits from the Dog
class. We also overrode the bark
method to provide a different implementation.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. This simplifies code and makes it more flexible.
def make_dog_bark(dog):
print(dog.bark())
# Create instances of different dog breeds
buddy = Dog("Buddy", "Golden Retriever")
spike = Bulldog("Spike", "Bulldog")
# Call the function with different dog objects
make_dog_bark(buddy) # Output: Woof!
make_dog_bark(spike) # Output: Woof! Woof!
Conclusion
In conclusion, this comprehensive guide has unraveled the intricacies of Python classes, leaving you equipped with a solid understanding of this essential concept in Python programming. By starting from the very basics and gradually progressing to more advanced topics like inheritance and polymorphism, we’ve ensured that both beginners and experienced developers can benefit from this tutorial. Python classes are the cornerstone of object-oriented programming in Python, enabling you to create structured, organized, and efficient code for a wide range of applications.
As you continue your programming journey, remember that Python classes are a powerful tool at your disposal. They provide a clear blueprint for modeling real-world entities in your code, making it more readable and maintainable. With the ability to create custom data types, inherit functionality from existing classes, and achieve polymorphism, Python classes empower you to write code that is both flexible and robust. So, whether you’re building a simple script or embarking on a complex software project, the knowledge you’ve gained about Python classes will be invaluable in your programming endeavors.
In the ever-evolving world of Python, grasping the nuances of Python classes is a crucial step toward becoming a proficient developer. With practice and exploration, you’ll find yourself harnessing the full potential of classes to create elegant, modular, and scalable Python programs. So, don’t hesitate to apply what you’ve learned, dive into coding projects, and continue expanding your Python skills. Happy coding with Python classes!