Absolutely! SQL joins are fundamental for querying data from multiple tables in a relational database. Here’s a comprehensive tutorial on SQL joins:
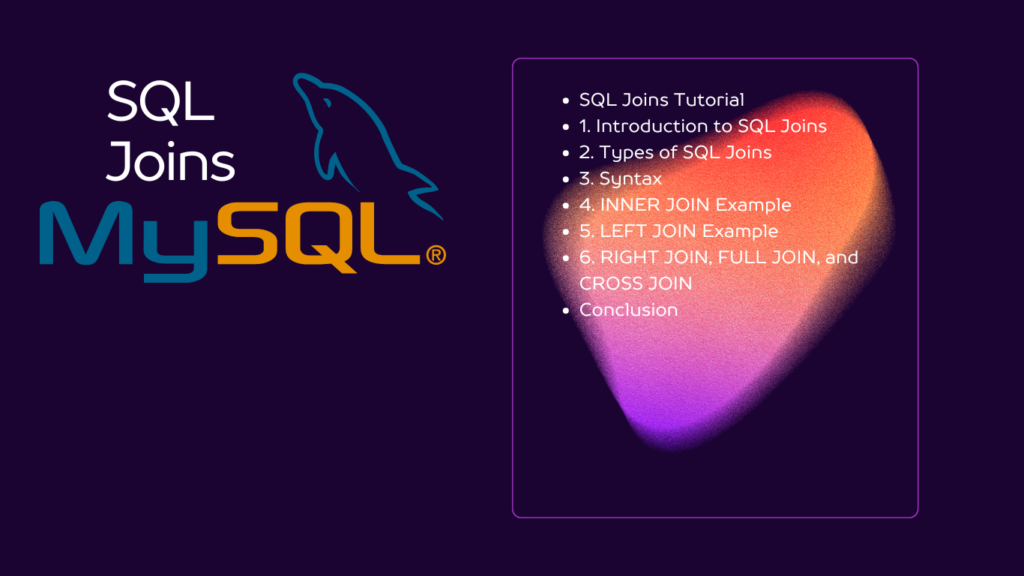
Table of Contents
SQL Joins Tutorial
1. Introduction to SQL Joins
SQL joins are used to combine rows from two or more tables based on a related column between them. The common columns are typically primary and foreign keys that establish relationships between tables.
2. Types of SQL Joins
There are several types of SQL joins:
- INNER JOIN: Returns records that have matching values in both tables.
- LEFT JOIN (or LEFT OUTER JOIN): Returns all records from the left table (first table mentioned) and the matched records from the right table (second table mentioned). If there is no match, NULL values are returned from the right side.
- RIGHT JOIN (or RIGHT OUTER JOIN): Returns all records from the right table and the matched records from the left table. Opposite of LEFT JOIN.
- FULL JOIN (or FULL OUTER JOIN): Returns all records when there is a match in either left or right table. If there is no match, NULL values are returned for the missing side.
- CROSS JOIN: Returns the Cartesian product of the two tables, meaning it combines every row of the first table with every row of the second table.
3. Syntax
SELECT columns
FROM table1
JOIN table2 ON table1.column = table2.column;
4. INNER JOIN Example
Suppose we have two tables: employees
and departments
.
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(50),
department_id INT
);
CREATE TABLE departments (
department_id INT PRIMARY KEY,
department_name VARCHAR(50)
);
INSERT INTO employees (employee_id, employee_name, department_id)
VALUES (1, 'John Doe', 1),
(2, 'Jane Smith', 2),
(3, 'Mike Johnson', 1);
INSERT INTO departments (department_id, department_name)
VALUES (1, 'IT'),
(2, 'HR');
To retrieve employees along with their department names:
SELECT e.employee_name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.department_id;
This will output:
employee_name | department_name
--------------|----------------
John Doe | IT
Jane Smith | HR
Mike Johnson | IT
5. LEFT JOIN Example
Now, let’s retrieve all employees regardless of whether they belong to a department:
SELECT e.employee_name, d.department_name
FROM employees e
LEFT JOIN departments d ON e.department_id = d.department_id;
Output:
employee_name | department_name
--------------|----------------
John Doe | IT
Jane Smith | HR
Mike Johnson | IT
Notice that even though Jane belongs to HR, all employees are listed.
6. RIGHT JOIN, FULL JOIN, and CROSS JOIN
The syntax for RIGHT JOIN, FULL JOIN, and CROSS JOIN is similar, just replace LEFT JOIN
with the respective keyword.
Conclusion
Understanding SQL joins is essential for manipulating data from multiple tables efficiently. Practice different types of joins to gain mastery.