let’s delve into the basics of SQL programming, specifically focusing on the HAVING
clause. SQL is a powerful language used for managing and manipulating relational databases. The HAVING
clause is used in conjunction with the GROUP BY
clause to filter the results of aggregate functions in SQL queries. It allows you to apply conditions to groups of rows, similar to how the WHERE
clause filters individual rows.
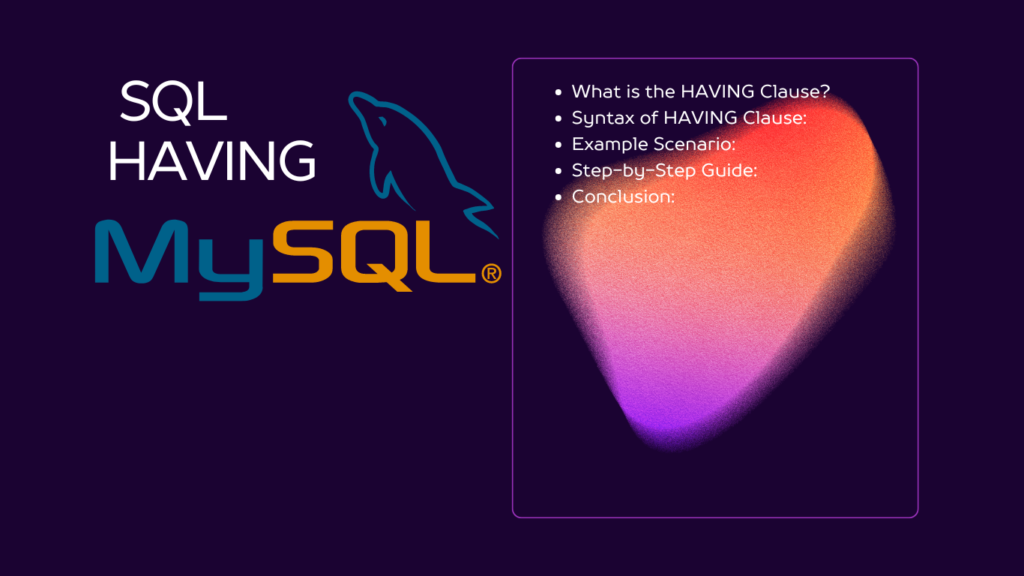
Table of Contents
What is the HAVING
Clause?
The HAVING
clause is typically used with the GROUP BY
clause to filter the results of aggregate functions applied to grouped rows. While the WHERE
clause filters individual rows before they are grouped, the HAVING
clause filters grouped rows after they have been aggregated.
Syntax of HAVING
Clause:
SELECT column1, aggregate_function(column2)
FROM table_name
GROUP BY column1
HAVING condition;
Example Scenario:
Let’s say we have a table named orders
with columns customer_id
, product_id
, and quantity
. We want to find customers who have purchased more than 10 products.
Step-by-Step Guide:
- Create Sample Data: Let’s create a sample table
orders
and insert some data into it:
CREATE TABLE orders (
customer_id INT,
product_id INT,
quantity INT
);
INSERT INTO orders (customer_id, product_id, quantity) VALUES
(1, 101, 5),
(1, 102, 15),
(2, 103, 8),
(2, 104, 20),
(3, 105, 12);
- Use
GROUP BY
andHAVING
: Now, let’s write a SQL query to find customers who have purchased more than 10 products:
SELECT customer_id, SUM(quantity) AS total_quantity
FROM orders
GROUP BY customer_id
HAVING SUM(quantity) > 10;
Explanation:
- We select the
customer_id
column and the sum ofquantity
for each customer. - We group the rows by
customer_id
. - We apply the
HAVING
clause to filter out groups where the sum ofquantity
is greater than 10.
- Expected Output:
+-------------+----------------+
| customer_id | total_quantity |
+-------------+----------------+
| 1 | 20 |
| 3 | 12 |
+-------------+----------------+
Explanation:
- Customer 1 has purchased a total of 20 products (5 + 15), which satisfies the condition.
- Customer 3 has purchased 12 products, which also satisfies the condition.
Conclusion:
The HAVING
clause is a powerful tool in SQL for filtering grouped data based on aggregate conditions. It allows you to apply conditions to the result of aggregate functions, enabling more complex data analysis and reporting. Practice using the HAVING
clause with different scenarios to strengthen your SQL skills.