The DROP
statement is used to delete objects from the database. These objects can be tables, databases, views, indexes, etc. In this tutorial, we will cover the following:
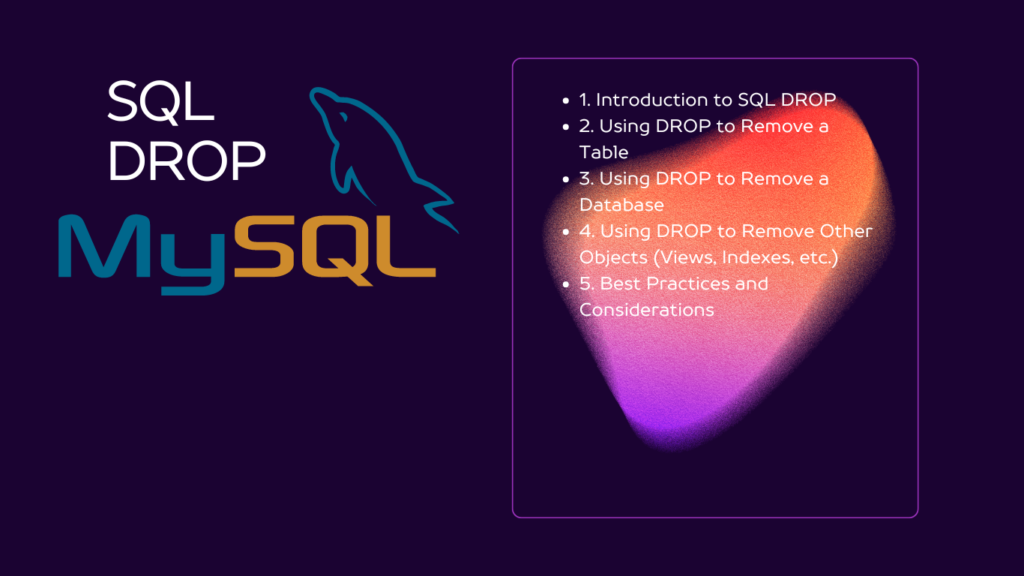
Table of Contents
1. Introduction to SQL DROP
The DROP
statement in SQL is a Data Definition Language (DDL) command. It is used to delete an existing database object completely from the database. When you use the DROP
statement, the specified object is permanently deleted along with all its data and structure.
Syntax:
DROP OBJECT_TYPE OBJECT_NAME;
OBJECT_TYPE
: The type of the object you want to delete (e.g., TABLE, DATABASE, VIEW, INDEX).OBJECT_NAME
: The name of the object you want to delete.
2. Using DROP to Remove a Table
Scenario: You have a table called Employees
that you want to delete from your database.
Step-by-Step Instructions:
- Create the
Employees
Table:CREATE TABLE Employees ( EmployeeID INT, FirstName VARCHAR(50), LastName VARCHAR(50), Position VARCHAR(50) );
- Verify the Table Exists:
SELECT * FROM Employees;
At this point, the table is empty but exists in the database. - Drop the
Employees
Table:DROP TABLE Employees;
- Verify the Table has been Dropped:
SELECT * FROM Employees;
This query will result in an error:ERROR 1146 (42S02): Table 'your_database.Employees' doesn't exist
, indicating that the table has been successfully dropped.
3. Using DROP to Remove a Database
Scenario: You have a database called CompanyDB
that you no longer need and want to delete.
Step-by-Step Instructions:
- Create the
CompanyDB
Database:CREATE DATABASE CompanyDB;
- Verify the Database Exists:
SHOW DATABASES LIKE 'CompanyDB';
This query will listCompanyDB
if it exists. - Drop the
CompanyDB
Database:DROP DATABASE CompanyDB;
- Verify the Database has been Dropped:
SHOW DATABASES LIKE 'CompanyDB';
This query will return an empty set, indicating that the database has been successfully dropped.
4. Using DROP to Remove Other Objects (Views, Indexes, etc.)
Scenario: You have a view called EmployeeView
and an index called EmployeeIndex
that you want to delete.
Step-by-Step Instructions:
- Create the
EmployeeView
View:CREATE VIEW EmployeeView AS SELECT EmployeeID, FirstName, LastName FROM Employees;
- Create the
EmployeeIndex
Index:CREATE INDEX EmployeeIndex ON Employees (LastName);
- Verify the View and Index Exist:
SHOW FULL TABLES IN your_database WHERE TABLE_TYPE LIKE 'VIEW'; SHOW INDEX FROM Employees WHERE Key_name = 'EmployeeIndex';
- Drop the
EmployeeView
View:DROP VIEW EmployeeView;
- Drop the
EmployeeIndex
Index:DROP INDEX EmployeeIndex ON Employees;
- Verify the View and Index have been Dropped:
SHOW FULL TABLES IN your_database WHERE TABLE_TYPE LIKE 'VIEW'; SHOW INDEX FROM Employees WHERE Key_name = 'EmployeeIndex';
Both queries should return empty sets, indicating that the view and index have been successfully dropped.
5. Best Practices and Considerations
- Backup Important Data: Always back up your data before performing any
DROP
operations, as they are irreversible. - Check Dependencies: Ensure that no other objects (like views, stored procedures, or foreign keys) depend on the object you’re dropping.
- Use
IF EXISTS
: To avoid errors if the object does not exist, useIF EXISTS
in yourDROP
statements.sql DROP TABLE IF EXISTS Employees; DROP DATABASE IF EXISTS CompanyDB; DROP VIEW IF EXISTS EmployeeView; DROP INDEX IF EXISTS EmployeeIndex ON Employees;
Summary
The DROP
statement in SQL is a powerful command used to delete database objects permanently. It is crucial to use it carefully to avoid accidental data loss. Always verify the existence of the object before dropping and ensure that you have backups of important data. By following the detailed instructions and best practices provided in this tutorial, you can safely and effectively use the DROP
statement in your SQL programming.