Absolutely, let’s delve into the basics of SQL programming, focusing specifically on the IN
operator. SQL, or Structured Query Language, is a powerful tool for managing and manipulating relational databases. The IN
operator allows you to specify multiple values in a WHERE clause, making it very handy for filtering data based on a set of conditions.
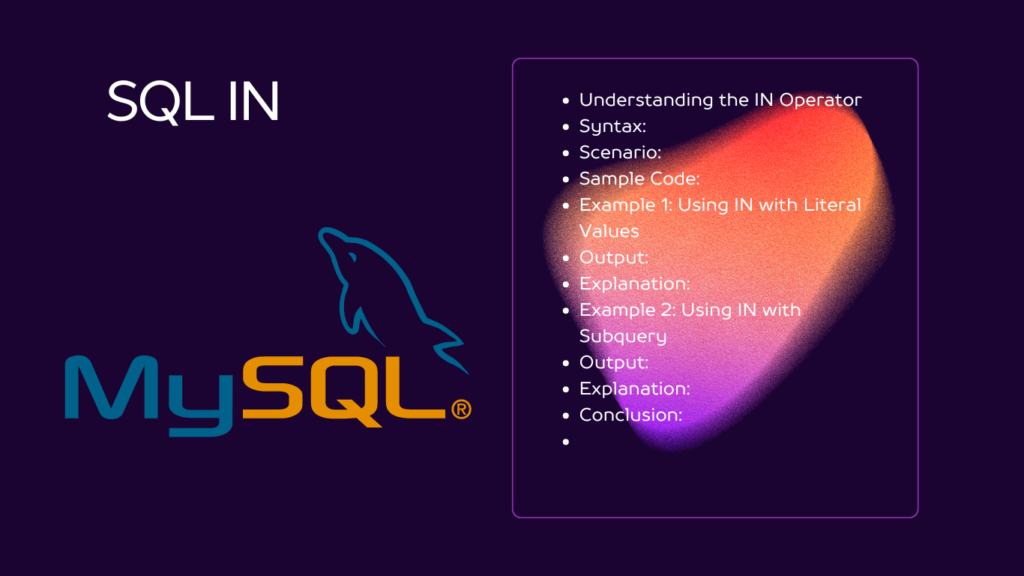
Table of Contents
Understanding the IN
Operator
The IN
operator allows you to specify multiple values in a WHERE clause. It is used to reduce the use of multiple OR conditions in a SELECT, INSERT, UPDATE, or DELETE statement.
Syntax:
SELECT column_name(s)
FROM table_name
WHERE column_name IN (value1, value2, ...);
Scenario:
Let’s say we have a database table named employees
, and we want to retrieve data for employees who belong to specific departments.
Sample Code:
Assume we have the following employees
table:
id | name | department |
---|---|---|
1 | Alice | HR |
2 | Bob | IT |
3 | Charlie | Sales |
4 | David | HR |
5 | Emily | IT |
Example 1: Using IN
with Literal Values
SELECT *
FROM employees
WHERE department IN ('HR', 'IT');
Output:
id | name | department |
---|---|---|
1 | Alice | HR |
2 | Bob | IT |
4 | David | HR |
5 | Emily | IT |
Explanation:
- The
IN
operator filters the rows where thedepartment
column value matches any of the specified values (‘HR’ or ‘IT’). - So, the output includes employees from both the ‘HR’ and ‘IT’ departments.
Example 2: Using IN
with Subquery
SELECT *
FROM employees
WHERE department IN (SELECT department FROM departments WHERE location = 'New York');
Output:
Assume we have a departments
table:
id | department | location |
---|---|---|
1 | HR | New York |
2 | IT | California |
3 | Sales | New York |
4 | Marketing | California |
id | name | department |
---|---|---|
1 | Alice | HR |
3 | Charlie | Sales |
Explanation:
- In this example, we are using a subquery to fetch department names from the
departments
table where the location is ‘New York’. - The
IN
operator then filters the rows from theemployees
table where thedepartment
column value matches any of the departments returned by the subquery. - So, the output includes employees who belong to the ‘HR’ or ‘Sales’ department, both of which are located in ‘New York’.
Conclusion:
The IN
operator is a powerful tool in SQL for filtering data based on multiple conditions. It simplifies queries and improves readability by reducing the need for multiple OR conditions. Whether used with literal values or subqueries, mastering the IN
operator is essential for efficient SQL programming.